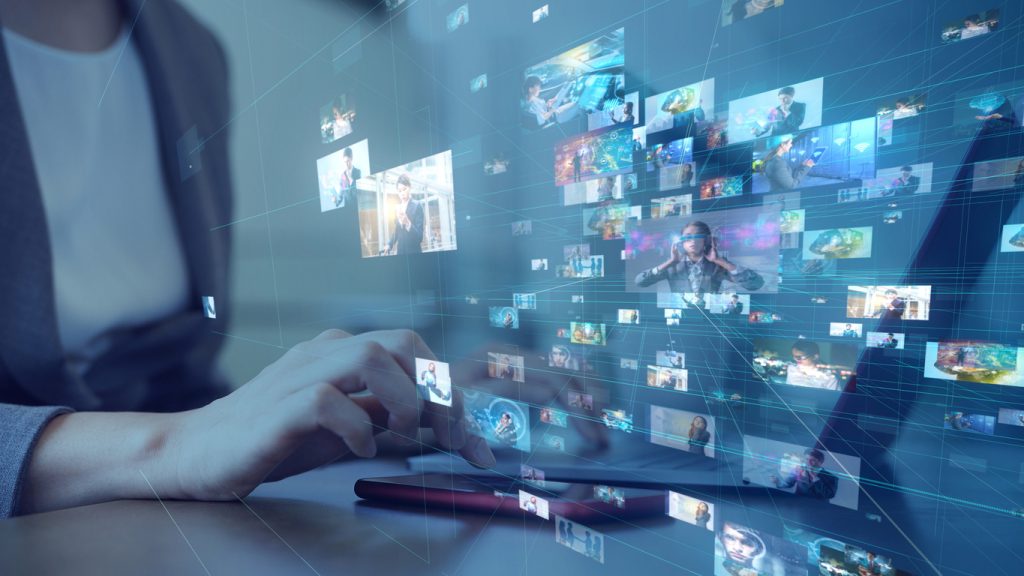
When building a website in Laravel (or any other PHP-based framework) where users will be uploading images, you will probably use a library to manipulate and process those images. With cell phone cameras capturing larger, higher-resolution images, raw images that are uploaded could quickly fill the available hard drive space and cause performance issues on the website.
While PHP has image processing libraries like GD and Imagick, these aren’t always user friendly. There are a number of third-party libraries that make image processing easier.
In this article we’ll be using Spatie Image, a PHP image processing library developed by Spatie, who creates a number of open source libraries for Laravel and PHP. It provides an easy-to-use interface for common image manipulations like resizing, cropping, and adding effects.
Installation and Basic Usage
Installation is done with a fairly simple composer command:
composer require Spatie Image
You can then use the Image
class to load and manipulate images.
use Spatie\Image\Image;
$image = Image::load('example.jpg)
->width(50)
->save();
Calling save
without any parameters will overwrite the existing file. If you want to save to a new file, then you would pass the new file path to the save
method. You can save to a different file format by changing the extension.
use Spatie\Image\Image;
$image = Image::load('example.jpg)
->save('example.png');
Resize an Image in PHP
Spatie Image provides a few different ways to resize an image.
Width and Height
The width
and height
functions take the desired dimensions in pixels as parameters and resize the image accordingly. The resized image will be contained within the given $width
and $height
dimensions respecting the original aspect ratio.
Image::load('example.png')
->width(300)
->height(250)
->save();
Resize
The resize function acts as a shortcut, allowing you to set width and height with a single method.
Image::load('example.png')
->resize(300, 250)
->save();
Fit
The Fit function lets you resize the image to a given width and height, but also accepts a Fit function as a parameter that gives you a bit more control as to how the image is resized.
Fit::Contain
resizes the image to fit within the width and height boundaries without cropping, distorting, or altering the aspect ratio.Fit::Max
resizes the image to fit within the width and height boundaries without cropping, distorting, or altering the aspect ratio, and will not increase the size of the image if it is smaller than the output size.Fit::Fill
resizes the image to fit within the width and height boundaries without cropping or distorting the image, and fills the remaining space with the background color (set using thebackground
function). The resulting image will match the constraining dimensions.Fit::Stretch
stretches the image to fit the constraining dimensions exactly. The resulting image will fill the dimensions, and will not maintain the aspect ratio of the input image.Fit::Crop
resizes the image to fill the width and height boundaries and crops any excess image data. The resulting image will match the width and height constraints without distorting the image.
Crop
By calling the crop
method, part of the image will be cropped to the given $width
and $height
dimensions (pixels). Use $cropMethod
to specify which part will be cropped out.
The following CropPosition
s are available on the enum: TopLeft
, Top
, TopRight
, Left
, Center
, Right
, BottomLeft
, Bottom
, BottomRight
.
Image::load('example.jpg')
->crop(250, 250, CropPosition::TopRight)
->save();
Manipulating the Image
Spatie Image provides a number of other methods to manipulate an image.
Adjustments
You can adjust the brightness, contrast, and gamma of the image.
Image::load('example.jpg')
->brightness(-20)
->save();
Effects
You can add effects like blur, pixelate, grayscale and sepia to the image.
Image::load('example.jpg')
->blur(20)
->save();
Watermarks
You can add another image as a watermark on the image.
Image::load('example.jpg')
->watermark('watermark.png', AlignPosition::center)
->save();
Text
You can add text to the image.
Image::load('example.jpg')
->text('Hello!')
->save();
Of course, you can combine all of these manipulations in a single call. For example, if we want to create a small, grayscale version of the image, we could do something like this:
Image::load('example.jpg')
->resize(64, 64)
->greyscale()
->brighten(20)
->save();
You can view the documentation here for more details about the library.